Calling app.use(express.json()) executes the middleware on every request made to our server. In your terminal, make sure youre still inside the express_app directory, and then run the following command: This will start your local server on PORT 3000. How to convert a file to zip file and download it using Node.js ? So Multer basically adds a file object or files object and a body object to the request object.
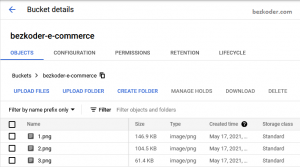
uploadFiles is the API controller.
The form-data library makes it easy to ensure your headers are set correctly by giving you a .getHeaders() method that will return headers appropriate to your form data. destination - It can also be given as a string (e.g. This is how multer binds the data whenever a form is submitted.
This variable now represents a readable stream of your file. Convert JSON file into CSV file and displaying the data using Node.js. '); id="fileupload" type="file" name="fileupload", id="upload-button" onclick="uploadFile()", Coffee Talk: Java, News, Stories and Opinions, How to format a Java String with printf example, How to format a Java int or long with printf example. If you have any questions, reach out to me on Twitter. Create a file with any name of your choice, we will call it app.js. Introduction: File uploading means a user from client machine requests to upload file to the server. To move the file to the folder of your choice, use the File System module,
The first thing it tries to do in the try block is create two variables: file and title. Express, you can easily grab all the inputs entered in the, to optimize your application's performance, Hyperledger Fabric: Develop permissioned blockchain smart contracts, How to market make and transact with Hashflow, How to create an AR experience with Unity and, How to build a blockchain charity or crowdsourcing platform.
Import file upload middleware at the beginning. The file object contains metadata related to the file.
Uploads to Node.js will go through Ajax, and thus create a full JavaScript file uploader with JavaScript running both on the client and the server. There are other modules in market but multer is very popular when it comes to file uploading. Im doing this tutorial on Node version 16.13.2.
The disk storage engine gives you full control over storing files to disk.
Load the express module using require() method and then set up a basic express server by writing the following code in app.js. The first step to upload files with Node is to get a simple web service working. Using this tutorial, I am seeing that my images files are correctly coming through in the request body within an array images (created on front end with each file from the file inputs filelist).
I hope you enjoyed this article and learned how to upload files directly from a Node.js app to Express. res.write('NodeJS File Upload Success! The reason line 3, where the file variable is created, is highlighted is because you need to replace the file path shown with the path to the file you want to upload. So this is how you can upload file in Node.js using multer module. If set to, Returns a HTTP 413 when the file is bigger than the size limit if true. So whenever you use multer, make sure you put multipart in form. Note that the files argument depends on the name of the input specified in formData. It is widely used and popular module for file uploading. Two key/value pairs are appended to the form: one for the file, and one for its title. action="http://localhost:80/upload" method="post" enctype="multipart/form-data". I hit a lot of dead ends on this route, and eventually decided to build an Express server that I would ultimately host on Heroku. In this example, because youre uploading only one file, youll use the single() method. How to install the previous version of node.js and npm ? It is your responsibility to provide a function that should return a complete filename with a file extension. Check out the Busboy documentation here. Below is a screenshot of the logged result: For reference, if you want to upload to a storage service like Cloudinary, you will have have to send the file directly from the uploads folder.
Then the code will look like this and will go in app.js: We can upload multiple files as well.
Multer is a Node.js middleware for handling multipart/form-data that makes the otherwise painstaking process of uploading files in Node.js much easier. By this, you can understand that multer is used to handle multipart/form-data. Django vs. Node.js: Pick the right web development New JavaScript runtime Bun challenges Deno, Node.js, .NET vs. Node.js: What they are, and which to choose, Optimizing Your Digital Workspaces?
If file uploading process successful, then you can go to the uploads folder and see your uploaded image as shown below. Come write articles for us and get featured, Learn and code with the best industry experts. Before using multer, we have to install it using npm.
We also built a small application using Node.js and Multer to see a file upload process.
Run the following command to install the multer package: From your text editor, open the index.js file found in the multipart_demo/express_app/routes folder. There is a very good module for working with file uploads, called "Formidable". This requires several third party libraries, and it was pretty difficult to make them all play nice together. The Formidable module can be downloaded and installed using NPM: After you have downloaded the Formidable module, you can include the module In this case, we have used multer({..}).single() which is used for uploading a single file. While using W3Schools, you agree to have read and accepted our. Well also explore Multer by building a mini app with a frontend and backend to test uploading a file. If you need the uploaded files to persist, you could store them in another directory on your server, so long as you specify the path in this object.
It accepts an options object, with dest property, which tells Multer where to upload the files. Read this first ! We will create a storage object using the diskStorage() method. Learn how to search logs with CloudWatch SaaS licensing can be tricky to navigate, and a wrong choice could cost you. Install Multer by running the following command in your terminal: To configure Multer, add the following to the top of server.js: Although Multer has many other configuration options, were only interested in thedest property for our project,which specifies the directory where Multer will save the encoded files. Using your favorite text editor, open the file called index.js that was created in your node_app folder. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. The array of files will be stored in req.files.
Difference between Fetch and Axios.js for making http requests. Use temp files instead of memory for managing the upload process. How could this post serve you better? On your backend, you should see the following: The code in the image above means that the req.body object is empty, which is to be expected. Now run the code by writing the following command in the terminal: After this, open the browser and type http://localhost:3000.
You can use custom regex to determine what to strip. It is similar to the popular Node.js body-parser, which is built into Express middleware for form submissions. How the single threaded non blocking IO model works in NodeJS ?
Please contact richardgirges [ at ] gmail.com if you're interested. The next step is to implement the Node.js JavaScript upload functionality. In order to follow along with this tutorial, youll need the following: In your terminal or command prompt, navigate to your general projects or development directory, and run the following commands: These commands will create a directory that holds both projects: your Node.js app and your Express API. Multer also creates a new object for multiple files, eitherreq.file or req.files, which holds information about those files. The 2 arguments to cb are: Now lets modify the variable a little bit. Both these functions take 3 arguments - the request object, the file object and a callback function (here, cb is callback function). How React Native Make Mobile App Development Simpler?
The title variable is where youll store the title of the file youre uploading - you can change this to whatever string value you like.
The path to this directory can be found in the "files" object, passed as the We will call it MulterApp. You will see the title of your file, along with the object representing it. The DIR const is passed to the destination, and it is responsible for storing the uploaded files. If youll recall, body-parser doesnt support multipart data. Lets get started!
For storing the files, you have to create 'public' folder and get inside of it and also create 'uploads' folder: The limits property handles the file size validation, in the below example we are seeing how to limit file size with multer.
Instead, well use Multer to parse the form. (1000000 bytes = 1MB).
Beneath these variables is where the form itself is created. Otherwise, the error message will be logged. At this point, the req object available in the endpoints callback function will be slightly different than what youre used to. However, Multer is returning an empty array for within req.files. I reckon you will like this tutorial and will share this tutorial other developers. How to trim a file extension from string using JavaScript ? In this case middleware uses console.log and adds Express-file-upload prefix for outputs. The first is by using the enctype attribute: The code above sends the form-data to the /upload_files path of your application. Web applications receive all different types of input from users, including text, graphical controls like checkboxes or radio buttons, and files, like images, videos, and other media. For my app, I only needed the file temporarily, so I set the destination to my servers /tmp folder. For a single file, use upload.single. At the end of this blog, you will be able to integrate the file uploads in your own apps. How to save an HTML 5 Canvas as an image on the server ? Learn to use the Internationalization API to convert and formate dates across time zones in JavaScript. It is mandatory to create a directory when you are using destination as a function. Applies uri decoding to file names if set true. This defines how long to wait for data before aborting. To do an Ajax and JavaScript file upload to Node.js, replace the form in the HTML page with these two lines: And add the following script before the end body tag: Save the index.html file and refresh the web browser. Below is a screenshot of the webpage so far: As you can see, the form we created takes two inputs, name and files. Ashley is a JavaScript Editor for the Twilio blog. What is Multipart Data?In general, when a form is submitted, browsers use application-xx-www-form-urlencoded content-type. Look to Analytics. Otherwise, if you are using destination as a string, multer will make sure that the directory is created for you.
Many organizations struggle to manage their vast collection of AWS accounts, but Control Tower can help. To keep your application performing well, you need to track various metrics. Youll see that if you stored the file to disk, there will be a path key - you can use this path to create another readable stream, like I needed to in order to upload the file to Google Drive. Further, open and update the following code in index.js file: For testing the Node File Upload REST API, you have to execute the below command from the project root: Start Postman application and here is the list of the REST APIs with their respective HTTP methods, that you can test now: Call HTTP POST request for uploading file: Here are the files we have uploaded, now you can check them on the public/uploads directory: Further, you will see how to fetch list of files using node REST API. The goal is to make you comfortable in building apps that can easily handle any file uploads. They both are functions. Multer adds a body object and a file or files object to the request object. 2. fileFilter - Set this to a function to control which files should be uploaded and which should be skipped. A multipart message will consist of text input and file input. Next, well install the required dependency, which for our purposes is Express: Next, create a server.js file and add the following code: Express contains the bodyParser object, which is a middleware for populating req.body with the submitted inputs on a form. We will be covering the following topics: Multer is a node.js middleware for handling multipart/form-data, which is primarily used for uploading files. To create one, navigate to the MulterApp directory in terminal, and write the following command: Answer a few questions (app name, git repo, etc) and youll be ready to roll. As we are not using any form tag or frontend therefore we will make requests and specify our form data from Postman only. With Multer, you can handle single or multiple files in addition to text inputs sent through a form. Now that we understand the importance of Multer, well build a small sample app to show how a frontend app can send three different files at once in a form, and how Multer is able to process the files on the backend, making them available for further use. If there are no errors, then an Upload complete message will be logged to the console. Practice Problems, POTD Streak, Weekly Contests & More! Editors note: This article was last updated 24 March 2022 to reflect updates to Node.js and the body-parser library. reaches the server. I hope you enjoyed this article! The multipart enctype setting on the form makes it possible to upload files to the server. The util module exposes the promisify() method, which is a common library of node.
In this project, we will store the uploaded files in a folder for simplicity. ', Angular 14 File Upload with Progress Bar Tutorial, Angular 14 Send and Get Route Parameters Example , How to Upload Excel to MySQL Database in Node JS, How to Build Google Places Autocomplete Address in Node Js, Node Js Import / Upload CSV File in MongoDB Database Tutorial. Setting this option to True turns on using temporary files instead of utilising RAM. If you don't provide any filename, each file will be given a random name without any file extension. Create a file named upload.js, and populate it with the following code: Open a command prompt in the same folder as the upload.js file and run the following command: [emailprotected]/c/upload-example $ node upload.js, As the command runs, open a browser and navigate to localhost:80/upload. // Note that this option available for versions 1.0.0 and newer. The body object contains the values of the text fields of the form, the file or files object contains the files uploaded via the form. Now let's add multer to our project. LogRocket is like a DVR for web and mobile apps, recording literally everything that happens while a user interacts with your app. We have also found out how to restrict file size from file uploading and how to restrict file extension for file uploading. The default value is application/x-www-form-urlencoded, which supports alphanumeric data.
Create a routes folder get into the directory, next create upload.route.js file by using the following command: Next, add the following code in routes/upload.route.js: In this step, you have to create an index.js file inside the root of your node project. Build a Svelte App that Uses the National Parks Service API to Plan Your Perfect Trip, Build a Serverless Call Routing Application with Time Zone Management in JavaScript, How to Call an AI Friend using GPT-3 with Twilio Voice and Functions, Converting and Formatting Dates and Time Zones with JavaScript, Create a Video App with SolidJS, Express, and Twilio Video.
Brian White for his stellar work on the Busboy Package and the connect-busboy Package, Gitgithub.com/richardgirges/express-fileupload, github.com/richardgirges/express-fileupload#readme. For instance, you can only upload png, jpg, and jpeg in this example; however, if the condition is not matched, then the error message will be displayed for the wrong file type. If you cant find her there, shes probably on a patio somewhere having a cup of coffee (or glass of wine, depending on the time). File upload is a common operation for any applications. Postman is an essential and fantastic tool if you are building rest API. In your terminal, navigate back to your parent directory, multipart-demo, and then into your Node.js app, with the following commands: Install the third-party dependencies youll need for this project: Youll use the form-data library to create a form with key/value pairs in your Node.js app. So we will install it one by one via npm(Node Package Manager). If no destination is given, the operating system's default directory for temporary files is used. When the operation is complete, it sends a Node.js File Upload Successmessage to the browser.
- 1" Black Iron Floor Flange
- Who Makes Eastwood Spray Guns
- Eileen West Heirloom Dream Chemise
- Cooling Tower Water Treatment Services
- Recipes Using Comstock Apple Pie Filling